binary tree python geeksforgeeks
Find all possible binary trees with given Inorder Traversal. Left view of a Binary Tree is set of nodes visible when tree is visited from Left side.
Bfs Vs Dfs For Binary Tree Geeksforgeeks
Platform to practice programming problems.
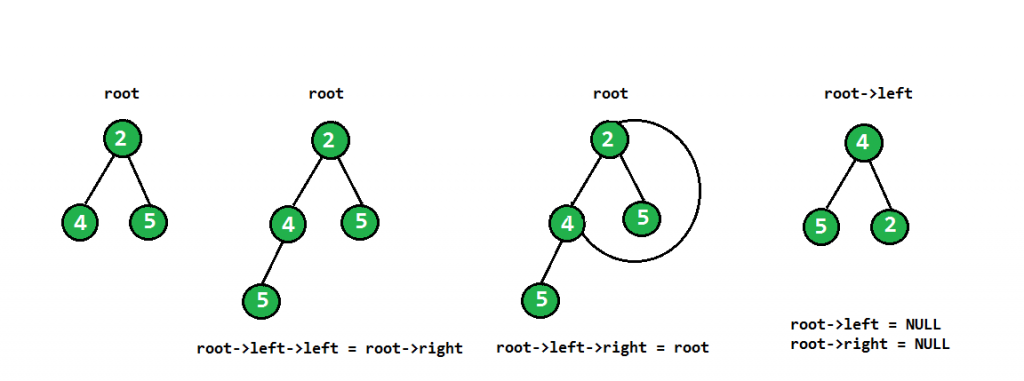
. In order to create a binary tree we first import the dstructure module create a BTree class object to initialize an empty binary tree and use the insert method to insert. We designate one node as root node and then add more nodes as child nodes. A binary tree is a tree that has at most two children.
Binary Search Tree is a node-based binary tree data structure which has the following properties. 2 3 The left subtree of a node contains only nodes with keys lesser than. Given a Binary Tree consisting of N nodes and a integer K the task is to find the depth and height of the node with value K in the Binary Tree.
A Computer Science portal for geeks. The node which is on the left of the Binary Tree is called Left-Child and the node. It means that each node in a binary tree can have either one or two or no children.
You dont need to read input or print anything. The task is to complete the function leftView which accepts root of the tree as argument. A complete binary tree of height h is a proper binary tree up to height h-1 and in the last level element are stored in left to right order.
We create a tree data structure in python by using the concept os node discussed earlier. 2 1 3 Output. It contains well written well thought and well explained computer science and programming articles quizzes and practicecompetitive.
A Binary Tree The height of the. Your task is to complete. QuizMock Test on binary search tree data structure.
Given a binary tree find its height. The quiz contains commonly asked questions on search insert and delete operations of BST. The depth of a node is the.
The tree is a hierarchical Data Structure. 1 2 3 Output. Print Postorder traversal from given Inorder and Preorder traversals.
Your task is to complete the function isBST which takes the root of the tree as a parameter and returns true if the given binary tree is BST else returns false. Solve company interview questions and improve your coding intellect. Replace each node in binary tree with the.
A binary tree is a tree data structure in which each node can have a maximum of 2 children.
Subtree With Given Sum In A Binary Tree Geeksforgeeks
Flip Binary Tree Geeksforgeeks
Introduction To Tree Data Structure And Algorithm Tutorials Geeksforgeeks
Tutorial On Binary Tree Geeksforgeeks
Diagonal Traversal Of Binary Tree Geeksforgeeks
Shortest Distance Between Two Nodes In Bst Geeksforgeeks
Difference Between Binary Tree And B Tree Geeksforgeeks
Product Of All Nodes In A Binary Tree Geeksforgeeks
Perfect Binary Tree Geeksforgeeks
Check If Two Trees Have Same Structure Geeksforgeeks
Print All Nodes At Distance K From A Given Node Geeksforgeeks
Find Mirror Of A Given Node In Binary Tree Geeksforgeeks
Count Of Root To Leaf Paths In A Binary Tree That Form An Ap Geeksforgeeks
Check Whether A Binary Tree Is A Complete Tree Or Not Set 2 Recursive Solution Geeksforgeeks
Serialize And Deserialize A Binary Tree Geeksforgeeks
Generate Complete Binary Tree In Such A Way That Sum Of Non Leaf Nodes Is Minimum Geeksforgeeks
Search A Node In Binary Tree Geeksforgeeks
Applications Of Bst Geeksforgeeks
Construct A Binary Tree In Level Order Using Recursion Geeksforgeeks